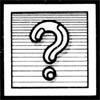
C. Regena
DATA
Statements
Data items are numbers or words that the computer works with. One way to get information into the computer is to use assignment statements such as LET A=4 or simply A=6 (the word LET is optional on most computer versions of BASIC). Another way to get information into the computer is to use READ statements with associated DATA statements. A DATA statement is ignored until a READ statement is encountered in the program, but then the READ continues through any DATA statements in order, picking off the information on these lines.
Greater Efficiency
Here's an example of a DATA statement with a READ statement that could replace seven LET assignment statements.
10 READ A,B,C,X,Y,Z,N$
20 DATA 5,3,2,4,1,8,BOB
30 PRINT A*B+C
When the computer comes to a READ statement which contains a variable name, it starts from the beginning of the program and looks for the first DATA statement. The first item in the DATA statement is assigned to the first variable in the READ statement. In this example, the value of 5 will be assigned to the variable A. Data items are separated by commas. The next variable to be read, B, is given the very next data item, 3. The next variable, C, is given the very next data item, 2. This process continues. But you won't see any results of the READ statement until you actually PRINT something. Line 30 uses some of the variables assigned to print a mathematical calculation.
The data items are always separated by commas and read in order, assigning each item to the variable name in the READ statement. You can have one or any number of variables in the READ statement. You can use numbers or strings. But you do need to be especially careful that the data items match up with the variables exactly as you want them to be assigned.
You can have any number of items in a DATA statement. When the computer finishes picking off the data items in one DATA statement, it goes to the very next DATA statement in the program. The number of items in the DATA statement does not have to match exactly the number of items in the READ statement, but eventually there do have to be enough data items for all the variables to be read. For example:
10 DATA 10,3,4
20 READ A,B,C,D,E,F,G
30 DATA 7,2,6,5
It doesn't matter whether the READ statement comes before the DATA statement or vice versa. In fact, they do not even need to be consecutive lines. In the example above, some of the data items are in line 10 before the READ statement and some are in line 30 after the READ statement. It's important to remember that the program ignores the DATA statements until the READ statement needs to look for data, and then that the DATA statements are used in the order they appear in the program.
Quite often you'll see a READ statement inside a FOR-NEXT loop to perform repeated operations, perhaps using subscripted variables or variables in an array. Here's an example using strings:
10 FOR C=1 TO 9
20 READ PL$(C),BB$(C)
30 NEXT C
40 DATA QUISENBERRY,P,SUND-
BERG,C
50 DATA BALBONI,1B,WHITE,2B,
BRETT,3B
60 DATA BIANCALANA,SS,LAW,LF
70 DATA WILSON,CF,BJACKSON,RF
The variable C is used as a counter in the loop. With each pass through the loop a player name PL$ and a position BB$ are read from the data. The DATA statements in lines 40-70 are kept short for this example, but you could put all the data into one or two statements to save memory
A Common Source Of Errors
For some programmers, DATA statements are the most common source of errors, especially if there are lots of items with commas or similar lines close together. If an error message refers to a line containing a READ statement, it's likely that an incorrect DATA statement is the real cause of the error. When you type DATA statements, you need to be especially careful with the placement of commas. If there are several commas together for null strings, be sure to type in the correct number of them. And avoid inadvertently typing a comma at the end of your data list. Also, period and comma keys are side-by-side on the keyboard, so it's easy to type a period in place of a comma, which may be difficult to spot in listings on the screen.
Note too that although your program might not always stop with an error message, something might not be working correctly. Again, a DATA statement could be at fault. For example, if you're reading in notes for music and the tune doesn't sound right, the numbers for the notes in the DATA statements would need to be checked.