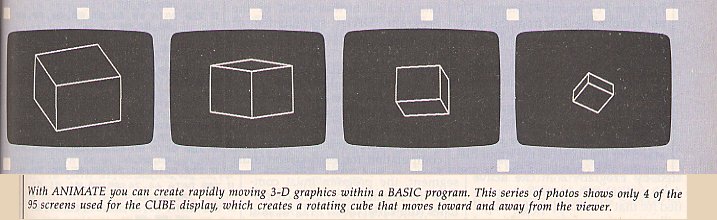
Easy IBM Full-Screen Animation
Paul W. CarlsonNow you can write BASIC programs with smooth, flicker-free animated displays that move at machine language speeds. For the IBM PC/PCjr. BASICA and a color/graphics card are required to use the program on the PC. Cartridge BASIC is required for the PCjr.
Full-screen animation is achieved by rapidly displaying a series of high-resolution screens on the video display. Producing realistic animation using BASIC is very difficult because of the time required to create the screen images. The creation of a high-resolution screen image usually consists of two processes repeated many times. First, the coordinates of the endpoints of a line segment are computed. Second, the line segment is displayed on the screen.
The method of animation presented here is unusual in that it completely separates the two processes. The computation of the coordinates of every line segment for every screen image is done by a BASIC program which writes the coordinates to disk as a binary (non-ASCII) file. This file of line segment coordinates is then input to a machine language program which displays the screens in rapid succession to produce the animation.
To begin, type in and save Program 1. Before you run this program, make sure you have a disk in the active drive with at least 60,000 bytes of available space. Now run Program 1; it creates a disk file named ANIMATE.OBJ containing the machine language animation routine. The DOS LINK utility must then be used to generate an executable version of this file. To do this, first exit DOS by typing SYSTEM and pressing Enter. Place a DOS system disk containing the file LINK.EXE in the active drive (check the master disk that came with your copy of DOS), type LINK, then press Enter. When you are prompted for the object modules, remove the DOS system disk and replace it with the disk containing ANIMATE.OBJ. At this point you should type ANIMATE„NUL,NUL and press Enter. After a minute or so the DOS prompt will reappear. Your disk now contains a new file named ANIMATE.EXE, the usable version of the machine language program that creates animated displays from the files produced by Programs 2 or 3.
A Rotating Demo
Now you are ready to type in and save Program 2 (this program can be saved on any disk). When you run the program, you will be prompted for an output filename. Enter any legal filename. Program 2 creates images of the word LOVE rotating in three-dimensions. After you press Enter, the program begins computing the line segment coordinates for each screen and writing them to the specified disk file. The display will show which screen is currently being computed. Program 2 computes 71 screens. Do not remove the disk from the drive until you see the message that the file is complete.
When the BASIC Ok prompt reappears, type SYSTEM and press Enter to exit to DOS. Put the disk containing ANIMATE.EXE in the active drive, then type ANIMATE and press Enter. When you are asked for the name of the input file, put the disk containing the file created by Program 2 in the active drive and enter the name you specified for that file. The disk drive light will go on for a few seconds, and then the animated image should appear on the screen. Press the Q key to terminate the display.
Once you have used Program 2 to create the animation data file, you won't need it again. However, before you delete it, notice that lines 430-520 also occur in Program 3. In fact, you'll find these lines in every program that you write that produces data files for the ANIMATE program. To save yourself a lot of typing, load Program 2 and delete all lines except 430-520; save the shortened program with a name you'll remember—you will probably use it as a template program many times.
To enter Program 3, first load the file containing lines 430-520 of Program 2. Then type in the other lines listed as Program 3 and save the file. At this point you should follow the same procedure as for Program 2. Program 3 computes 95 screens. The computation for each screen takes longer than those in Program 2 because of computations to remove hidden lines from the display. Now run the animate program using this data file as input. You will see a rotating cube repeatedly coming toward and going away from you (see photos).
Make Your Own Art
Writing your own programs with ANIMATE is not difficult. Just follow these steps:
- Load the template file containing the lines 430-520.
- All DIM statements and initialization of variables should be performed prior to line 430. If there is not enough room in the program to do this, you can GOSUB to a routine located further down in the program. DATA statements, of course, can be placed anywhere in the program.
- The variable NUMSCNS should be assigned a value equal to the number of screens to be displayed. This assignment must also be done prior to line 430.
- The subroutine that does the computation for each screen must begin at line 1000. For each line segment, the program must compute the segment endpoint coordinates (the variables X1, Y1, X2, and Y2) and execute a GOSUB 500.
The ANIMATE program can handle up to 4000 line segments. This means that the number of screens times the number of line segments per screen cannot exceed 4000.
Programs 2 and 3 both produced 3-D images, but this doesn't mean that you need to know 3-D geometry to create impressive displays. Two-dimensional animation, when it's fast and smooth, can be truly spectacular as well.
For instructions on entering these listings, please refer to "COMPUTE!'s Guide to Typing In Programs" in this issue of COMPUTE!.
Program 1: ANIMATE.OBJ File Maker
KN 10 T=0:OPEN "ANIMATE.OBJ" FOR OUTPUT AS 1 KL 20 FOR J=1 TO 1076:READ A$:N=VAL("&H"+A$) FA 30 T=T+N:PRINT#1,CHR$(N);:NEXT:CLOSE 1 PL 40 IF T=84992! THEN PRINT"FILE SUCCESSFULLY CREATED!":END LM 50 PRINT CHR$(7);"***** ERROR IN DATA STATEMENTS *****":END BE 100 DATA 80,03,00,01,41,3B,96,11,00,00 OD 110 DATA 04,43,53,45,47,04,44,53,45,47 BI 120 DATA 04,53,53,45,47,D6,98,07,00,60 OM 130 DATA E1,01,02,01,01,1B,98,07,00,60 NB 140 DATA 9D,BF,03,01,01,A0,98,07,00,74 LM 150 DATA 80,00,04,01,01,67,A0,0C,00,02 AA 160 DATA 00,00,80,40,20,10,08,04,02,01 JL 170 DATA 53,A2,0F,00,02,08,00,00,40,01 EF 180 DATA 00,01,00,00,00,02,00,00,01,A2 BO 190 DATA 0F,00,02,08,80,A0,1F,01,00,01 PG 200 DATA 00,00,00,02,00,00,02,A0,1A,00 GC 210 DATA 02,48,BF,00,00,00,00,00,00,00 BE 220 DATA 00,00,00,00,00,00,00,00,00,00 JH 230 DATA 00,00,00,14,00,29,A2,0E,00,02 NH 240 DATA 5E,BF,14,00,01,00,01,00,00,00 OC 250 DATA 01,20,FA,A0,2F,00,02,72,BF,00 NK 260 DATA 00,45,6E,74,65,72,20,69,6E,70 DF 270 DATA 75,74,20,66,69,6C,65,20,6E,61 MM 280 DATA 6D,65,3A,20,24,0A,0D,46,69,6C DP 290 DATA 65,20,6E,6F,74,20,66,6F,75,6E II 300 DATA 64,24,49,A0,01,01,01,00,00,1E MI 310 DATA 33,C0,50,B8,00,00,8E,D8,B8,00 DG 320 DATA 06,B7,07,B9,00,00,BA,4F,18,CD DJ 330 DATA 10,33,D2,B7,00,B4,02,CD,10,8D IO 340 DATA 16,00,00,B4,09,CD,21,8D,16,00 DL 350 DATA 00,B4,0A,CD,21,B7,00,8A,1E,00 GC 360 DATA 00,C6,87,00,00,00,8D,16,00,00 NK 370 DATA B0,00,B4,3D,CD,21,73,09,8D,16 IA 380 DATA 00,00,B4,09,CD,21,CB,A3,00,00 IN 390 DATA 8B,1E,00,00,8D,16,00,00,52,B9 PG 400 DATA 80,00,B4,3F,CD,21,5A,81,C2,80 OG 410 DATA 00,3D,00,00,75,EE,B8,06,00,CD PI 420 DATA 10,E8,00,00,8D,1E,00,00,8B,07 FJ 430 DATA 3D,9D,FF,74,2F,3D,19,FC,75,05 FI 440 DATA E8,00,00,EB,EB,A3,00,00,83,C3 JK 450 DATA 02,8B,07,A3,00,00,83,C3,02,8B OO 460 DATA 07,A3,00,00,83,C3,02,8B,07,A3 BK 470 DATA 00,00,83,C3,02,53,E8,00,00,5B GC 480 DATA EB,CA,E8,00,00,B4,06,B2,FF,CD NE 490 DATA 21,3C,71,74,04,3C,51,75,1B,32 BB 500 DATA FF,B8,00,06,33,C9,BA,4F,18,CD LO 510 DATA 10,B8,00,02,33,DB,33,D2,CD,10 NL 520 DATA B8,02,00,CD,10,CB,E8,00,00,83 HP 530 DATA C3,02,EB,96,1E,06,8C,D8,8E,C0 PB 540 DATA 8D,3E,00,00,B8,00,B8,8E,D8,33 DN 550 DATA F6,B9,A0,1F,FC,F3,A5,07,1F,C3 IB 560 DATA 06,B8,45,9C,94,00,C8,05,00,02 BB 570 DATA 02,9D,BF,C4,20,00,02,02,74,BF EH 580 DATA C4,28,00,02,02,5C,BF,C4,32,00 FL 590 DATA 02,02,5D,BF,C4,36,00,02,02,5E EI 600 DATA BF,C4,3B,00,02,02,5E,BF,C4,47 LB 610 DATA 00,02,02,8C,BF,C4,4F,00,02,02 OF 620 DATA 72,BF,C4,53,00,02,02,72,BF,C4 BO 630 DATA 57,00,02,02,08,00,84,71,00,01 KO 640 DATA 01,E1,00,C4,75,00,02,02,08,00 NG 650 DATA 84,84,00,01,01,0F,01,C4,89,00 MB 660 DATA 02,02,48,BF,C4,91,00,02,02,4A LE 670 DATA BF,C4,99,00,02,02,4C,BF,C4,A1 BF 680 DATA 00,02,02,4E,BF,84,A8,00,01,01 PK 690 DATA 22,01,84,AE,00,01,01,FB,00,84 FA 700 DATA DA,00,01,01,0F,01,C4,E9,00,02 LD 710 DATA 02,08,80,1B,A0,E8,00,01,FD,00 DK 720 DATA 00,B8,8E,C0,B9,A0,1F,33,FF,8D DB 730 DATA 36,00,00,FC,F3,A5,07,C3,06,8C PB 740 DATA D8,8E,C0,B9,A0,1F,8D,3E,00,00 JI 750 DATA 33,C0,FC,F3,AB,07,C3,06,8C,D8 JC 760 DATA 8E,C0,BE,01,00,BF,01,00,8B,16 JB 770 DATA 00,00,2B,16,00,00,7D,04,F7,DF MA 780 DATA F7,DA,89,3E,00,00,8B,0E,00,00 FP 790 DATA 2B,0E,00,00,7D,04,F7,DE,F7,D9 AE 800 DATA 89,36,00,00,3B,CA,7D,08,BE,00 GL 810 DATA 00,87,CA,EB,04,90,BF,00,00,89 QJ 820 DATA 36,00,00,89,3E,00,00,8B,C2,D1 LJ 830 DATA E0,A3,00,00,2B,C1,8B,D8,2B,C1 DC 840 DATA A3,00,00,8B,36,00,00,8B,3E,00 DJ 850 DATA 00,41,56,53,8B,C7,8A,E0,25,FE GH 860 DATA 01,D1,E0,D1,E0,D1,E0,8B,D8,80 NE 870 DATA E7,07,D1,E0,D1,E0,03,D8,8D,06 GK 880 DATA 00,00,03,D8,8B,C6,D1,F8,D1,F8 DG 890 DATA D1,F8,03,D8,81,E6,07,00,8A,84 QP 900 DATA 00,00,26,0A,07,26,88,07,5B,5E LI 910 DATA 83,FB,00,7D,11,03,36,00,00,03 KC 920 DATA 3E,00,00,03,1E,00,00,E2,B3,EB MM 930 DATA 0F,90,03,36,00,00,03,3E,00,00 EJ 940 DATA 03,1E,00,00,E2,A2,07,C3,82,9C JP 950 DATA 99,00,C4,0B,00,02,02,08,80,C4 EJ 960 DATA 1C,00,02,02,08,80,C4,32,00,02 OD 970 DATA 02,4E,BF,C4,36,00,02,02,4A,BF KD 980 DATA C4,40,00,02,02,52,BF,C4,44,00 HI 990 DATA 02,02,4C,BF,C4,48,00,02,02,48 OP 1000 DATA BF,C4,52,00,02,02,50,BF,C4,65 NM 1010 DATA 00,02,02,54,BF,C4,69,00,02,02 HN 1020 DATA 56,BF,C4,70,00,02,02,58,BF,C4 BH 1030 DATA 79,00,02,02,5A,BF,C4,7D,00,02 CL 1040 DATA 02,48,BF,C4,81,00,02,02,4A,BF BD 1050 DATA C4,A0,00,02,02,08,80,C4,B4,04 MP 1060 DATA 02,02,C4,C5,00,02,02,54,BF,C4 CP 1070 DATA C9,00,02,02,56,BF,C4,CD,00,02 IA 1080 DATA 02,58,BF,C4,D6,00,02,02,50,BF PM 1090 DATA C4,DA,00,02,02,52,BF,C4,DE,00 PG 1100 DATA 02,02,5A,BF,38,90,0E,00,00,01 MH 1110 DATA 07,41,52,52,59,53,43,4E,FB,00 BK 1120 DATA 00,3D,90,0C,00,00,01,05,45,52 IN 1130 DATA 41,53,45,0F,01,00,DE,90,0E,00 JO 1140 DATA 00,01,07,4D,45,4D,4C,49,4E,45 EK 1150 DATA 22,01,00,30,90,0E,00,00,01,07 CH 1160 DATA 53,43,4E,41,52,52,59,E1,00,00 HE 1170 DATA 57,8A,02,00,00,74
Program 2: LOVE File Maker
There's something odd about this program which I can't verify. The loops here go from 0 to 11 instead of 1 to 11. An interval of 0 to 11 means 12 items and the arrays are dimensioned for only 11. So, if the checksums don't match or BASIC gives you an error, change lines 20 and 1000 to loop from 1 to 11.
DJ 10 DIM BX(11),BY(11),EX(11),EY(11) PH 20 FOR N=0 TO 11:READ BX(N),BY(N),EX(N),EY(N):NEXT DC 30 DATA -22,3,-22,-3,-22,-3,-14,-3 OA 40 DATA -10,3,-10,-3,-10,-3,-2,-3 EL 50 DATA -2,-3,-2,3,-2,3,-10,3 JG 60 DATA 2,3,6,-3,6,-3,10,3 FG 70 DATA 22,3,14,3,14,3,14,-3 OJ 80 DATA 14,-3,22,-3,20,0,14,0 GA 90 CX=320:CY=100:A=6.2831853# CF 100 NUMSCNS=71 JN 430 INPUT"OUTPUT FILE NAME";F$:OPEN F$ FOR OUTPUT AS 1 EE 440 PRINT"COMPUTING SCREEN NUMBER: "; MB 450 FOR SCRN=1 TO NUMSCNS:PRINT SCRN; NH 460 GOSUB 1000 IE 470 PRINT#1,CHR$(157);CHR$(255);:NEXT SCRN FO 480 PRINT#1,CHR$(25);CHR$(252):CLOSE 1:PRINT BJ 490 PRINT"ANIMATION DATA FILE ";CHR$(34);F$;CHR$(34);" IS COMPLETE":END CL 500 PRINT#1,CHR$(INT(X1) AND 255);CHR$(INT(X1/256));CHR$(INT(Y1));CHR$(0); KA 510 PRINT#1,CHR$(INT(X2) AND 255);CHR$(INT(X2/256));CHR$(INT(Y2));CHR$(0); MD 520 RETURN HF 1000 FOR N=0 TO 11 KL 1010 ZE=-BX(N)*SIN(A)+30 PO 1020 X1=100*BX(N)*COS(A)/ZE+CX:Y1=-100*BY(N)/ZE+CY MP 1030 ZE=-EX(N)*SIN(A)+30 ML 1040 X2=100*EX(N)*COS(A)/ZE+CX:Y2=-100*EY(N)/ZE+CY II 1050 GOSUB 500 DI 1060 NEXT N:A=A-8.726646E-02 JA 1070 RETURN
Program 3: CUBE File Maker
BF 1 ' PROGRAM 3 OH 2 ' MH 10 DIM V(8,3),SV(8,2),S(6,5),N(6,3),E(12,3) FE 20 FOR I=1 TO 8:FOR J=1 TO 3:READ V(I,J):NEXT J,I AG 30 FOR I=1 TO 6:FOR J=1 TO 5:READ S(I,J):NEXT J,I KM 40 DATA 40,40,-40,40,40,40,40,-40,40,40,-40,-40 PJ 50 DATA -40,-40,-40,-40,-40,40,-40,40,40,-40,40,-40 LE 60 DATA 1,2,3,4,1,1,8,7,2,1,8,5,6,7,8 NE 70 DATA 5,4,3,6,5,2,7,6,3,2,4,5,8,1,4 KP 90 CX=320:CY=100:TH=.2:PH=.8:PPD=2000:DIST=20000 HH 100 NUMSCNS=95 JN 430 INPUT"OUTPUT FILE NAME";F$:OPEN F$ FOR OUTPUT AS 1 EE 440 PRINT"COMPUTING SCREEN NUMBER: "; MB 450 FOR SCRN=1 TO NUMSCNS:PRINT SCRN; NH 460 GOSUB 1000 IE 470 PRINT#1,CHR$(157);CHR$(255);:NEXT SCRN FO 480 PRINT#1,CHR$(25);CHR$(252):CLOSE 1:PRINT BJ 490 PRINT"ANIMATION DATA FILE ";CHR$(34);F$;CHR$(34);" IS COMPLETE":END CL 500 PRINT#1,CHR$(INT(X1) AND 255);CHR$(INT(X1/256));CHR$(INT(Y1));CHR$(0); KA 510 PRINT#1,CHR$(INT(X2) AND 255);CHR$(INT(X2/256));CHR$(INT(Y2));CHR$(0); MD 520 RETURN KC 1000 S1=SIN(TH):C1=COS(TH):S2=SIN(PH):C2=COS(PH) PP 1010 FOR I=1 TO 8:X=V(I,1):Y=V(I,2):Z=V(I,3):SX=-X*S1+Y*C1 FL 1020 SY=-X*C1*C2-Y*S1*C2+Z*S2:SZ=-X*S2*C1-Y*S2*S1-Z*C2+DIST IB 1030 SV(I,1)=PPD*(2.67*SX/SZ)+CX:SV(I,2)=—PPD*(SY/SZ)+CY:NEXT IO 1040 FOR I=1 TO 6:F=S(I,1):G=S(I,2):H=S(I,3):U1=V(G,1)-V(F,1):U2=V(G,2)-V(F,2) FL 1050 U3=V(G,3)-V(F,3):V1=V(H,1)-V(F,1):V2=V(H,2)-V(F,2):V3=V(H,3)-V(F,3) GJ 1060 N(I,1)=U2*V3-V2*U3:N(I,2)=U3*V1-V3*U1:N(I,3)=U1*V2-V1*U2:NEXT NI 1070 XE=DIST*S2*C1:YE=DIST*S2*S1:ZE=DIST*C2:M=1 JO 1080 FOR I=1 TO 6:E2=S(I,1):WX=XE-V(E2,1):WY=YE-V(E2,2):WZ=ZE-V(E2,3) MH 1090 IF (N(I,1)*WX+N(I,2)*WY+N(I,3)*WZ)<=0 THEN 1140 DI 1100 E1=S(I,1):FOR J=2 TO 5:E2=S(I,J):FOR K=1 TO M KH 1110 IF E(K,1)=E2 AND E(K,2)=E1 THEN E(K,3)=2:GOTO 1130 EP 1120 NEXT K:E(M,1)=E1:E(M,2)=E2:E(M,3)=1:M=M+1 BH 1130 E1=E2:NEXT J CB 1140 NEXT I:FOR I=1 TO 12:IF E(I,3)=0 THEN 1160 CH 1150 J=E(I,1):K=E(I,2):X1=SV(J,1):Y1=SV(J,2):X2=SV(K,1):Y2=SV(K,2):GOSUB 500 PC 1160 NEXT:TH=TH+6.544985E-02:PH=PH+6.544985E-02:IF SCRN<48 THEN PPD=PPD+583.3:RETURN IA 1170 PPD=PPD-583.3:RETURN