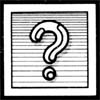
Tom R Halfhill, Editor
More
String-Slicing
Last month we saw how you can copy pieces of character strings using the LEFT$ and RIGHT$ functions found in versions of Microsoft BASIC. For even more flexibility, most Microsoft-style BASICs include a third function for extracting sections of strings. Called MID$ ("mid-string"), this function lets you copy a section from the middle of a string.
The basic format is MID$ (string$, n1,n2), where string$ is a string variable or literal string; n1 is a number representing the beginning character position of the substring you want to extract; and n2 is a number representing the number of characters in the substring you want to extract. For example:
10 A$="JAMES FENIMORE COOPER"
20 PRINT MID$(A$,7,8)
30 B$=MID$(A$,11,4)
40 PRINT B$
50 PRINT A$
When you run this program, the result is:
FENIMORE
MORE
JAMES FENIMORE COOPER
Line 20 prints the eight characters starting at position seven in A$, resulting in the substring FENIMORE. (Remember that spaces count as characters.) Lines 30 and 40 do much the same thing, but copy the four characters starting at position 11 into the string variable B$ before printing them out. This method is useful if you need to print B$ later in your program or manipulate B$ in some other way. Line 50 shows that the MID$ function, like LEFT$ and RIGHT$, does not disturb the original contents of A$.
MID$ is handy for so many different things that it's hard to come up with a generalized example. It can even be used to replace LEFT$ and RIGHT$-for instance, MID$(A$,1,10) is equivalent to LEFT$(A$,10), and MID$(A$,LEN(A$)-9,LEN(A$)) is the same as RIGHT$(A$,10). One useful application of MID$ is to store a bunch of short strings as a single long string, then pick out the substring you want with MID$. For example, let's say you're writing some sort of program that needs to print out the months of the year, perhaps as labels for a budget or chart. You could abbreviate the names of the months as equallength substrings within one large string, like this:
10 M$="JANFEBMARAPRMAYJUNJUL
AUGSEPOCTNOVDEC"
Now suppose that the numeric variable M contains the number of the month you need to print out maybe as a result of an INPUT statement:
20 PRINT "WHICH MONTH TO
PRINT";
30 INPUT M
40 PRINT MID$(M$,M*3-2,3)
Depending on the user's response (1 for January, 2 for February, etc.), line 40 prints out the proper month name. Or you could print out all the months with a loop-FOR M=1 TO 12:PRINT MID$(M$,M*3-2,3) :NEXT M.
Storing all the months in a single string and extracting the one you want with MID$ is more efficient than using 12 separate strings for the same purpose. It's also more efficient in some ways than a string array (a subject we'll cover in a future column).
Atari And TI Strings
There are no LEFT$, RIGHT$, or MID$ functions in TI BASIC or the Atari BASIC found on Atari 400/800, XL, and XE computers. These BASICs handle strings a little differently than Microsoft BASIC does. (Note that Microsoft BASIC is available on cartridge for Atari computers, and some BASICs available from independent suppliers also support Microsoft-style strings.)
TI BASIC's statement for segmenting strings is SEG$. It works exactly like MID$ in Microsoft BASIC-the statement B$=SEG$ (A$,11,4) is equivalent to B$=MID$(A$,11,4). You can simulate LEFT$ with a statement in the form SEG$(string$,nl,n2), where string$ is the string you wish to manipulate, n1 is the starting character position of the segment within the string, and n2 is the number of characters you wish to print or copy. For example, the statement B$=LEFT$(A$,6) can be replaced with B$=SEG$(A$,1,6).
Simulating RIGHT$ is a bit more complicated. You need a statement in the form SEG$(string $,LEN(string$)-n1,n2), where n2 is the number of characters you wish to print or copy, and n1 is n2-1. For example, B$=RIGHT$(A$,6) can be replaced with B$=SEG$ (A$,LEN(A$)-5,6).
Atari BASIC requires the same sort of manipulations. To print or copy any substring in Atari BASIC, simply specify the starting and ending character positions of the substring within the larger string. To translate B$=LEFT$(A$,6), use B$=A$(1,6). To simulate RIGHT$, use a statement in the form string$(LEN(string$)-n,LEN(string$)), where string$ is the string you're manipulating and n is the number of characters you wish to print or copy minus one. For instance, to translate B$=RIGHT$(A$,6), use B$=A$(LEN(A$)-5,LEN(A$)). To simulate MID$, use the statement string$(nl,n2), where n1 is the starting character position (just like MID$), and n2 equals n1 plus the number of characters you wish to print or copy minus one. Thus, the Microsoft statement B$=MID$ (A$,11,4) is translated as B$=A$ (11,14).