DIAMOND
DROP
DROP
Matt Giwer
Catch the falling diamonds-if you can. This fast-action game is easy to play and uses very little memory. Originally written for the Atari (with paddle), other versions are included for the TI-9914A (with Extended BASIC) and the VIC and 64.
"Diamond Drop" is a game that requires good judgment and quick reflexes. It's fast, easy to play, and will fit into even the smallest Atari. The game uses both player/missile graphics and the Atari's fast string handling. The game plays quickly in BASIC with no machine language routines and uses less than 7K of RAM.
Four rows of diamonds will appear at the top of the screen. At the bottom, you'll see five catching trays, which are controlled by your paddle. As the diamonds drop, position your trays to catch them. Each diamond is worth ten points. If you miss, you lose one tray. If you complete one row, you get a 100-point bonus. Finish all four rows and you get a 250-point bonus. When you have lost all of your trays, the high score is recorded on the left of the screen, and you start again.
You won't be able to anticipate a dropping pattern because the subroutine at line 20000 generates a random sequence of two-digit numbers that will not repeat. Each number appears only once within the string.
The routine starts off with AA$ (line 20012), which contains the numbers 05 through 34. (These are the column numbers for the POSITION in structions.) The G LOOP then picks two of these pairs of numbers randomly and exchanges their positions within AA$. Thirty exchanges within this string of thirty pairs of numbers work well for this game.
Understanding The Program
Line 2 sends us immediately to line 30000 where the subroutine turns on the P/M Graphics and draws the trays at 30282. For a real challenge, change the POKE in line 30210 to 0.
Line 80 DIMensions the strings for the order of dropping the diamonds, four small strings for shuffling, and a string for scoring.
Line 100 names the frequently called subroutines for ease of program development and modification.
The subroutine at line 1000 initializes the variables and screen with a new set of four rows of diamonds. (Diamonds are CTRL ".").
Lines 2010 through 2190 comprise an infinite (because of the STEP size) control loop for the main program execution. Within this loop is the nested J LOOP (lines 2040 and 2090). This loop moves the diamond from the top of the screen to the bottom in line 2051. The second POSITION and ? put a blank in the previous position of the diamond as it moves down. Line 2080 contains the collision register for Player 0 and directs execution to the subroutine for catching (line 5400). Upon return from the subroutine, POKE HITCLR 53278 clears the collision registers.
Subroutine CATCH sets FLG =1. If the flag has not been set, line 2100 slides the diamond off to the right of the screen. The program is then directed to subroutine MISS.
The 5100 lines decrement the ROW and give a bonus and GOSUB SCORE. If all four rows are gone, the program then moves to NLEVEL.
The 5300 lines give a bonus, increase the score, then initialize the variables and reset the screen with GOSUB 1000.
The 5400 lines simply remove the diamond, give a buzz, and increment the score.
The 5500 lines increment the score by 10. Note that the bonuses have been 90 and 240, since each time this subroutine is called, 10 is added. Line 5520 prints the score vertically on the right side of the screen.
The 5600 lines remove one of the trays, provide a sound effect, and test for all trays being gone.
The 5700 lines determine if the new score is a high score, print it on the left side of the screen, erase the old score, and ask for another game. Pressing the trigger sets up a new game.
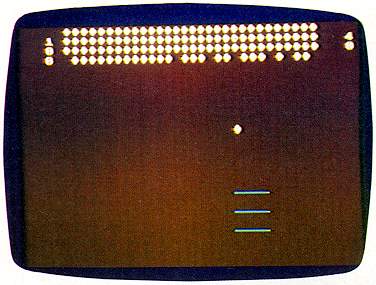
"Diamond Drop" on the Atari.
Program 1: Diamond Drop-Atari Version
2 GOSUB 30000
80 DIM AA$(60),B1$(2),C1$(2),B2$(2),
C2$(2),SCR$(8)
99 MM=0
100 NROW=5100:NLEVEL=5300:CATCH=5400
:SCORE=5500:MISS=5600:TEND=5700
900 GOSUB 1000:GOTO 2000
1000 REM SETUP
1009 REM 5 TO 34
1010 POSITION 5,0:? "


1020 POSITION 5,1:? "


1030 POSITION 5,2:? "


1040 POSITION 5,3:? "


1050 GOSUB 20000:ROW=4
1900 RETURN
2000 REM CONTROL LOOP
2010 FOR ZYX=1 TO 2 STEP 0
2020 A=VAL(AA$(I,I+1))
2022 POKE PLX,PADDLE(0)
2030 I=I+2
2040 FOR J=ROW TO 22
2042 POKE PLX_,PADDLE(0)
2051 POSITION A,J:"'";:POSITION A,
J-1:? " ";
2080 IF PEEK(53252)<>0 THEN GOSUB CA
TCH:POKE 53278,0
2090 NEXT J
2100 IF FLG=0 THEN FOR FF=A TO 35:PO
SITION FF,22:? "'";:POSITION FF
-1,22:? " ";:POKE PLX,PADDLE(0)
:NEXT FF
2102 POSITION
2110 IF FLG=0 THEN GOSUB MISS
2180 IF 1:>60 THEN GOSUB NROW:GOSUB 2
0000
2188 FLG=0
2190 NEXT ZYX
5100 REM NROW NEW ROW
5110 ROW=ROW-1:SC=SC+90:GOSUB SCORE
5120 IF ROW=0 THEN GOSUB NLEVEL
5190 RETURN
5300 REM NLEVEL NEW LEVEL
5310 SC=SC+240:GOSUB SCORE
5350 GOSUB 1000
5390 RETURN
5440 REM CATCH
5410 POSITION A,J:? " ";:J=24:FLG=1
5420 SOUND 0,A,2,14
5430 Q=1^1
5440 SOUND 0,0,0,0
5450 GOSUB SCORE
5490 RETURN
5500 REM SCORE
5510 SC=SC+10:SCR$=STR$(SC)
5520 FOR FF=1 TO LEN(SCR$):SOUND 0,2
00-20*FF,10,14:POSITION 38,FF:?
SCR$(FF,FF):SOUND 0,0,0,0:NEXT
FF
5590 RETURN
5600 REM MISS THE BALL
5610 POKE MYPMBASE+135+MM,0
5615 FOR JKJ=14 TO 0 STEP -2:FOR JKk;
=200 TO 50 STEP -50:SOUND 0,JKK
,10,JKJ:NEXT JKK:NEXT JKJ
5620 MM=MM+15:IF MM+135=210 THEN GOS
UB TEND
5690 RETURN
5700 REM TEND THE END
5710 IF SC>HSC THEN HSC=SC
5720 SCR$=STR$(HSC)
5730 FOR FF=1 TO LEN(SCR$):SOUND 0,2
00-FF*10,10,14:POSITION 3,FF:?
SCR$(FF,FF):Q=1^1:SOUND 0,0,0,0
:NEXT FF
5740 SC=0:FOR FF=1 TO 10:POSITION 38
,FF:? " ";:NEXT FF
5750 POSITION 2,10:? "ANOTHER GAME?"
:Q=1^1
5752 IF PTRIG(0) THEN 5752
5754 POSITION 2,10:? "{13 SPACES}";:P
OKE 77,3
5760 FOR III=MYPMBASE+135 TO MYPMBAS
E+200 STEP 15:POKE III,255:NEXT
III
5770 GOSUB 1000:MM=0
5790 RETURN
20000 REM RND GENERATOR
20002 I=1
20012 AA$="0506070809101112131415161
718192021222.324252627282930313
23334"
20040 FOR G=0 TO 30
20050 M=INT(30*RND(0)+2)*2-3
20051 N=INT(30*RND(0)+2)*2-3
20060 B1$=AA$(M,M+1):C1$=AA$(N,N+1)
20070 B2$=C1$:C2$=B1$
20080 AA$(M,M+1)=B2$:AA$(N,N+1)=C2$
20081 POKE PLX,PADDLE(0)
20090 NEXT G
20092 A=VAL(AA$(1,2))
20099 RETURN
22000 FOR I=MYPMBASE TO MYPMBASE+255
:POKE I,0:NEXT I:STOP
30000 REM SETUP
30010 GRAPHICS 0:POKE 752,1
30200 REM PM SETUP
30204 POKE 53277,3:REM GRACTL PLAY?M
ISS
30206 POKE 559,62:REM DMACTL,1LINE,P
LAY,MIS,NORM FIELD
30208 POKE 54279,(PEEK(106)-12):REM
12PAGE RESERVE
30210 POKE 53256,1:REM PLAY SIZES
30212 POKE 623,8:REM PRIORITY PF OVE
R PL
30214 MYPMBASE=256*(PEEK.(106)-12):RE
M NEW PM BASE
30230 POKE 704,150
30232 POKE 710,16:POKE 709,29
30276 PLX=53248
30282 FOR I=MYPMBASE+135 TO MYPMBASE
+200 STEP 15:POKE I,255:NEXT I
30283 POKE PLX,PADDLE(0)
30285 RETURN
Program 2:
Diamond Drop - VIC Version, Part 1
by Eric Brandon, Programming Assistant
3 POKE55,177:POKE56,27:CLR
4 POKE36879,93
5 TI$="000000"
9 PRINT"{CLR}{BLU}{4 DOWN}SETTING UP ...
{3 DOWN}"
10 I=7089
15 PRINT"WAIT"STR$(25-VAL(TI$))" SECONDS
{UP}"
20 READA:IFA=256THEN40
30 POKEI,A:I=I+1:GOTO15
40 PRINT"{CLR){5 DOWN}{RED}{RVS}NOW LOAD
ING GAME... {OFF}{BLU}"
50 REM FOR DISK USERS, TAKE THE WORD "RE
M" OUT OF LINE 60
55 PRINT"{2 DOWN}"
60 REM PRINT" {UP}LOAD"CHR$(34)"DIAMONDS2
.VIC"CHR$(34)",8"
70 PRINT"{4 UP}"
80 POKE 198,1:POKE631,131:NEW
7089 DATA 120,169,27,141,21,3,169
7097 DATA 200,141,20,3,88,169,9
7105 DATA 141,253,29,169,0,141,250
7113 DATA 29,96,173,255,29,141,252
7121 DATA 29,172,253,29,169,32,153
7129 DATA 205,31,200,169,160,174,251
7137 DATA 29,153,205,31,200,202,208
7145 DATA 249,169,32,153,205,31,206
7153 DATA 252,29,208,3,76,174,28
7161 DATA 172,253,29,169,32,153,161
7169 DATA 31,200,169,160,174,251,29
7177 DATA 153,161,31,200,202,208,249
7185 DATA 169,32,153,161,31,200,206
7193 DATA 252,29,208,3,76,174,28
7201 DATA 172,253,29,169,32,153,117
7209 DATA 31,200,169,160,174,251,29
7217 DATA 153,117,31,200,202,208,249
7225 DATA 169,32,153,117,31,200,206
7233 DATA 252,29,240,123,172,253,29
7241 DATA 169,32,153,73,31,200,169
7249 DATA 160,174,251,29,153,73,31
7257 DATA 200,202,208,249,169,32,153
7265 DATA 73,31,200,206,252,29,240
7273 DATA 91,172,253,29,169,32,153
7281 DATA 29,31,200,169,160,174,251
7289 DATA 29,153,29,31,200,202,208
7297 DATA 249,169,32,153,29,31,200
7305 DATA 206,252,29,240,59,172,253
7313 DATA 29,169,32,153,241,30,200
7321 DATA 169,160,174,251,29,153,241
7329 DATA 30,200,202,208,249,169,32
7337 DATA 153,241,30,200,206,252,29
7345 DATA 240,27,172,253,29,169,32
7353 DATA 153,197,30,200,169,160,174
7361 DATA 251,29,153,197,30,200,202
7369 DATA 208,249,169,32,153,197,30
7377 DATA 200,165,197,201,21,208,13
7385 DATA 173,253,29,201,1,240,24
7393 DATA 206,253,29,76,211,28,201
7401 DATA 22,208,14,173,253,29,24
7409 DATA 109,251,29,201,21,240,3
7417 DATA 238,253,29,238,250,29,173
7425 DATA 250,29,205,249,29,240,3
7433 DATA 76,191,234,169,0,141,250
7441 DATA 29,169,206,133,251,169,31
7449 DATA 133,252,160,0,185,206,31
7457 DATA 41,127,201,32,208,74,200
7465 DATA 192,21,208,242,160,0,177
7473 DATA 251,201,81,240,37,201,207
7481 DATA 240,33,201,90,240,29,200
7489 DATA 192,22,208,237,56,165,251
7497 DATA 233,22,133,251,176,2,198
7505 DATA 252,166,251,208,220,166,252
7513 DATA 224,30,208,214,76,191,234
7521 DATA 170,152,24,105,22,168,138
7529 DATA 145,251,152,56,233,22,168
7537 DATA 169,32,145,251,32,154,29
7545 DATA 76,14,29,169,32,153,206
7553 DATA 31,169,150,141,11,144,169
7561 DATA 175,141,12,144,169,15,141
7569 DATA 14,144,169,200,133,251,160
7577 DATA 128,162,8,142,15,144,232
7585 DATA 224,15,208,248,200,208,243
7593 DATA 230,251,208,239,169,14,141
7601 DATA 15,144,169,0,141,14,144
7609 DATA 141,12,144,141,11,144,160
7617 DATA 21,185,0,30,201,81,240
7625 DATA 11,136,208,246,169,1,141
7633 DATA 254,29,76,191,234,169,32
7641 DATA 153,0,30,76,191,234,152
7649 DATA 72,180,10,185,0,30,201
7657 DATA 57,208,9,169,48,153,0
7665 DATA 30,136,76,158,29,185,0
7673 DATA 30,24,105,1,153,0,30
7681 DATA 104,168,96,174,255,29,202
7689 DATA 142,255,29,232,169,206,133
7697 DATA 251,169,31,133,252,56,165
7705 DATA 251,233,44,133,251,176,2
7713 DATA 198,252,202,208,242,160,0
7721 DATA 177,251,201,160,240,4,200
7729 DATA 76,218,29,174,251,29,169
7737 DATA 32,145,251,200,202,208,250
7745 DATA 96,96,256
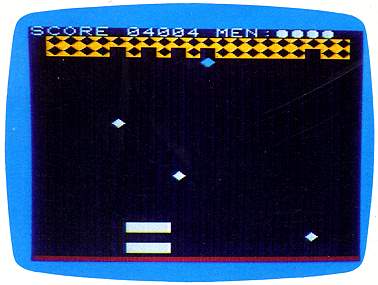
VIC version of "Diamond Drop."
Program 3:
Diamond Drop - VIC Version, Part II
by Eric Brandon, Programming Assistant
5 POKE 36879,14
10 PRINT"{CLR}{WHT}"TAB(5)"DIAMOND DROP"
20 PRINT"{2 DOWN}{YEL}{2 SPACES}CATCH TH
E DIAMONDS{2 SPACES}BEFORE THEY ";
30 PRINT"TOUCH THE GROUND. YOU HAVE FIVE
40 PRINT"CHANCES.
45 PRINT"{2 DOWN}{WHT}{4 SPACES}L - MOVE
LEFT
46 PRINT"{DOWN}{4 SPACES}; - MOVE RIGHT
{YEL}"
50 PRINT"{3 DOWN}[<6>] {RVS}HIT ANY KEY
TO BEGIN"
60 GETA$:IFA$=""THEN60
65 GOSUB 1000
70 PRINT"{CLR}{WHT}SCORE 00000 MEN:QQQQ"
71 SPEED = 7673
72 PADDLES=7679
73 FLAG=7678: POKE FLAG,0
74 WIDTH = 7675
75 POKE PADDLES,6 : POKE WIDTH,W : POKE
SPEED,10-S
78 ROW(6)=81:ROW(5)=81:ROW(4)=207:ROW(3)
=207:ROW(2)=90:ROW(1)=90
80 PRINT" {YEL}{RVS}";:FORI=1TO20:PRINT"
Z";:NEXT:PRINT"{OFF} ";
85 PRINT" {YEL){RVS} ";:FORI=1TO20:PRINT"
Z";:NEXT:PRINT"{OFF} ";
90 PRINT" {CYN}{RVS} ";:FORI=1TO20:PRINT"
P";:NEXT:PRINT"{OFF} ";
95 PRINT" {CYN}{RVS} "; :FORI=lTO20: PRINT"
P";:NEXT:PRINT"{OFF} ";
100 PRINT" {OFF}[c71";:FORT=1TO20:PRINT
"W";:NEXT:PRINT" ";
102 PRINT" {OFF)97~";:FORI=1TO20:PRINT
"W";:NEXT:PRINT" ";
105 PRINT"{WHT}";
109 REM 22 SPACES IN NEXT LINE
110 FORI=1TO14:PRINT"{22 SPACES}";:NEXT
120 PRINT"{HOME}";
130 FOR I=8164 TO 8185: POKE I,248:POKE
I+30720,2:NEXT
140 IF PEEK(789)<>27THENSYS 7089
150 FOR ROW = 6 TO 1STEP-1:FOR CHAR=1 TO
20
155 FOR K=1 TO 600-CHAR*10+(6-ROW)*20-50
*(9-PEEK(SPEED)):NEXT
157 IF PEEK(FLAG) THEN 2000
160 P=RND(1)*20+1
170 IF PEEK(7680+ROW*22+P)=32THEN160
180 POKE 7680+ROW*22+P,ROW(ROW)
190 NEXTCHAR
191 POKE36878,15
192 POKE36876,249
193 FORH=75TO15STEP-1.5:POKE 36878,H/5:N
EXTH
194 POKE36878, 0
197 IF ROW >1 THENSYS 7610
200 NEXTROW
201 FOR K=1 TO 300:NEXTK
205 IF PEEK(SPEED)>2 THEN POKE SPEED,PEE
K(SPEED)-1
206 IF PEEK(SPEED)=2 AND PEEK(WIDTH)>lTH
ENPOKEWIDTH,PEEK(WIDTH)-1
207 POKE PADDLE,6
210 PRINT"{HOME}{DOWN}";
220 GOTO 80
999 END
1000 PRINT"{CLR}{7 SPACES}DIFFICULTY
{4 SPACES}{5 DOWN}"
1010 INPUT"{WHT}SPEED (1-9)(YEL)
{3 RIGHT}5{3 LEFT}";S
1015 IF S>9 OR S<l THEN 1010
1020 INPUT"{3 DOWN}{WHT}WIDTH (1-6){YEL}
{3 RIGHT}3{3 LEFT}";W
1030 IF W>6 OR W<l THEN 1020
1040 RETURN
2000 PRINT"{HOME}{10 DOWN}{6 SPACES}
{YEL}GAME OVER"
2005 PRINT"{UP}HIT SPACE TO CONTINUE"
2010 POKE 198,0
2020 GETA$:IFA$<>" "THEN2020
2030 RUN 65
VIC-20/64 Version
Notes Eric Brandon, Programming Assistant To insure fast action, both the VIC and 64 versions of "Diamond Drop" are written predominantly in machine language. BASIC is used only to print instructions, set up the display, select the skill level, and initiate the "drop." The game display starts with six rows of objects at the top of the screen and a stack of six catching trays at the bottom. As the objects begin to drop, you must use the L and; keys to maneuver the trays and catch the objects. To make play more challenging, one tray disappears whenever the last ball drops from a row. Thus, you have only one tray with which to catch objects from the last row. When all the objects have dropped, you start again with six rows of objects and six trays. Play continues until a total of five objects hit the ground. The VIC version is in two parts (Programs 2 and 3) so that it can run on the unexpanded VIC. Cassette users should type in Program 2 and SAVE it to tape, then type in Program 3 and SAVE it on the same tape immediately following Program 2. Disk users should type in Program 2, omitting the word REM in line 60, and SAVE it to disk. Program 3 should then be typed in and SAVEd to the same disk with the filename "DIAMONDS2.VIC". If the tape or disk copies are prepared in this manner, then Program 2 will cause Program 3 to LOAD and RUN automatically. Since the DATA statements of Program 2 (VIC version) and Program 4 (64 version) comprise the machine language program for the game, it is essential that they be typed correctly. Be sure to SAVE a copy of the program before you attempt to RUN it, since an error in typing may cause your computer to "lock up," forcing you to turn the power off to recover. If Diamond Drop fails to RUN properly, the problem will most likely be a mistyped number somewhere in the DATA statements, so check carefully. |
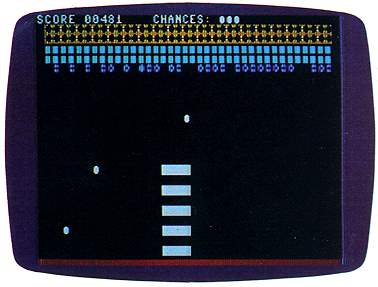
The diamonds are falling from the sky in "Diamond Drop,"
64 version
Program 4: Diamond Drop - 64 Version
by Eric Brandon, Programming Assistant
5 POKE 53280,12:POKE53281,0
7 IF PEEK(49152)<>120THENGOSUB49000
9 SYS 49745
10 PRINT"{CLR}{WHT}"TAB(13)"DIAMOND DROP
"
20 PRINT"{5 DOWN}{YEL}{5 SPACES}CATCH TH
E DIAMONDS BEFORE THEY
30 PRINT"{DOWN}{5 SPACES}TOUCH THE GROUN
D. YOU HAVE FIVE
40 PRINT"{DOWN}{5 SPACES}CHANCES.
45 PRINT"{2 DOWN}{WHT}{13 SPACES}L - MOV
E LEFT
46 PRINT"{13 SPACES}; - MOVE RIGHT{YEL}"
50 PRINT"{5 DOWN}[<6>]{9 SPACES}{RVS}HIT
ANY KEY TO BEGIN"
60 GETA$:IFA$=""THEN60
65 GOSUB 1000
70 PRINT"{CLR}{WHT}SCORE 00000{4 SPACES}
CHANCES: QQQQ "
71 SPEED = 53241
72 PADDLES=12*4096+4095
73 FLAG=12*4096+4094 : POKE FLAG,0
74 WIDTH = 12*4096+15*256+15*16+11
75 POKE PADDLES,6 : POKE WIDTH,W : POKE
SPEED,10-S
78 ROW(6)=81:ROW(5)=81:ROW(4)=207:ROW(3)
=207:ROW(2)=90:ROW(1)=90
80 PRINT" {YEL}{RVS}";:FORI=1TO38:PRINT"
Z";:NEXT:PRINT"{OFF} ";
85 PRINT" {YEL}{RVS}";:FORI=1TO38:PRINT"
Z";:NEXT:PRINT"{OFF} ";
90 PRINT" {CYN}{RVS}";:FORI=1TO38:PRINT"
P";:NEXT:PRINT"(OFF} ";
95 PRINT" {CYN}{RVS}";:FORI=1TO38:PRINT"
P";:NEXT:PRINT"{OFF} ";
100 PRINT" {OFF}[<7>]";:FORT=1TO38:PRINT
"W";:NEXT:PRINT" ";
102 PRINT" {OFF}[<7>]";:FORI=1TO38:PRINT
"W";:NEXT:PRINT" ";
105 PRINT"{WHT}";
109 REM 40 SPACES IN NEXT LINE
110 FORI=1TO17:PRINT"{40 SPACES}";:NEXT
120 PRINT"{HOME}";
130 FOR I=1984 TO 2023 : POKE I,248:POKE
I+54272,10:NEXT
140 IF PEEK(789)<>12*16THENSYS 12*4096
150 FOR ROW = 6 TO 1STEP-1:FOR CHAR=1 TO
38
155 FOR K=1 TO 600-CHAR*10+(6-ROW)*20-50
*(9-PEEK(SPEED)):NEXT
157 IF PEEK(FLAG) THEN 2000
160 P=RND(1)*38+1
170 IF PEEK(1024+ROW*40+P)=32THEN160
180 POKE 1024+ROW*40+P,ROW(ROW)
190 NEXTCHAR
191 SYS 49745
192 FORQ=1TO2:POKE54296,05 :POKE54277,5:
POKE54278, 218
193 POKE 54273,150 :POKE54272,139:POKE54
276,17
194 FORT=1TO50:NEXT:POKE54276,16:FORT=IT
O10:NEXT
195 NEXTQ
197 IF ROW >1 THENSYS 49691
200 NEXTROW
201 FOR K=1 TO 300:NEXTK
205 POKE PADDLE,6
206 IF PEEK(SPEED)=2 AND PEEK(WIDTH)>l T
HEN POKE WIDTH,PEEK(WIDTH)-1
207 IF PEEK(SPEED)>2 THEN POKE SPEED,PEE
K(SPEED)-1
210 PRINT"(HOME}(DOWN}";
220 GOTO 80
999 END
1000 PRINT"{CLR}{7 SPACES}DIFFICULTY
{4 SPACES}{5 DOWN}"
1010 INPUT"{WHT}SPEED (1-9){YEL}
{3 RIGHT}5{3 LEFT}";S
1015 IF S>9 OR S<l THEN 1010
1020 INPUT"{3 DOWN}{WHT}WIDTH OF PADDLES
(1-9){YEL}{3 RIGHT}4{3 LEFT}";W
1030 IF W>9 OR W<1 THEN 1020
1040 RETURN
2000 PRINT"{HOME}{10 DOWN}{2 SPACES}
{YEL}GAME OVER - HIT SPACE TO CONTI
NUE"
2010 POKE 198,0
2020 GETA$:IFA$<>" "THEN2020
2030 RUN 65
49000 PRINT"{WHT}{CLR}{2 DOWN}LOADING MA
CHINE LANGUAGE ...{3 DOWN}":TI$="00
0000"
49005 I=49152
49007 PRINT"READY IN"STR$(29-VAL(TI$))"
SECONDS {UP}"
49010 READ A:IF A=256 THEN RETURN
49020 POKE I,A:I=I+1:GOTO 49007
49152 DATA 120,169,192,141,21,3,169
49160 DATA 29,141,20,3,88,169,18
49168 DATA 141,253,207,169,0,141,250
49176 DATA 207,141,247,207,141,248,207
49184 DATA 96,173,255,207,141,252,207
49192 DATA 172,253,207,169,32,153,151
49200 DATA 7,200,169,160,174,251,207
49208 DATA 153,151,7,200,202,208,249
49216 DATA 169,32,153,151,7,206,252
49224 DATA 207,208,3,76,3,193,172
49232 DATA 253,207,169,32,153,71,7
49240 DATA 200,169,160,174,251,207,153
49248 DATA 71,7,200,202,208,249,169
49256 DATA 32,153,71,7,200,206,252
49264 DATA 207,208,3,76,3,193,172
49272 DATA 253,207,169,32,153,247,6
49280 DATA 200,169,160,174,251,207,153
49288 DATA 247,6,200,202,208,249,169
49296 DATA 32,153,247,6,200,206,252
49304 DATA 207,240,123,172,253,207,169
49312 DATA 32,153,167,6,200,169,160
49320 DATA 174,251,207,153,167,,6,200
49328 DATA 202,208,249,169,32,153,167
49336 DATA 6,200,206,252,207,240,91
49344 DATA 172,253,207,169,32,153,87
49352 DATA 6,200,169,160,174,251,207
49360 DATA 153,87,6,200,202,208,249
49368 DATA 169,32,153,87,6,200,206
49376 DATA 252,207,240,59,172,253,207
49384 DATA 169,32,153,7,6,200,169
49392 DATA 160,174,251,207,153,7,6
49400 DATA 200,202,208,249,169,32,153
49408 DATA 7,6,200,206,252,207,240
49416 DATA 27,172,253,207,169,32,153
49424 DATA 183,5,200,169,160,174,251
49432 DATA 207,153,183,5,200,202,208
49440 DATA 249,169,32,153,183,5,200
49448 DATA 165,197,201,42,208,13,173
49456 DATA 253,207,201,1,240,24,206
49464 DATA 253,207,76,40,193,201,50
49472 DATA 208,14,173,253,207,24,109
49480 DATA 251,207,201,39,240,3,238
49488 DATA 253,207,238,250,207,173,250
49496 DATA 207,205,249,207,240,3,76
49504 DATA 49,234,169,0,141,250,207
49512 DATA 169,112,133,251,169,7,133
49520 DATA 252,160,0,185,152,7,41
49528 DATA 127,201,32,208,74,200,192
49536 DATA 39,208,242,160,0,177,251
49544 DATA 201,81,240,37,201,207,240
49552 DATA 33,201,90,240,29,200,192
49560 DATA 40,208,237,56,165,251,233
49568 DATA 40,133,251,176,2,198,252
49576 DATA 166,251,208,220,166,252,224
49584 DATA 4,208,214,76,49,234,170
49592 DATA 152,24,105,40,168,138,145
49600 DATA 251,152,56,233,40,168,169
49608 DATA 32,145,251,32,251,193,76
49616 DATA 99,193,169,32,153,152,7
49624 DATA 32,81,194,169,15,141,24
49632 DATA 212,169,17,141,5,212,169
49640 DATA 213,141,6,212,169,2,141
49648 DATA 3,212,169,100,141,2,212
49656 DATA 169,5,141,1,212,169,135
49664 DATA 141,0,212,169,65,141,4
49672 DATA 212,160,0,162,0,142,32
49680 DATA 208,232,208,250,200,208,247
49688 DATA 169,12,141,32,208,169,64
49696 DATA 141,4,212,160,39,185,0
49704 DATA 4,201,81,240,11,136,208
49712 DATA 246,169,1,141,254,207,76
49720 DATA 49,234,169,32,153,0,4
49728 DATA 76,49,234,152,72,160,10
49736 DATA 185,0,4,201,57,208,9
49744 DATA 169,48,153,0,4,136,76
49752 DATA 255,193,185,0,4,24,105
49760 DATA 1,153,0,4,104,168,96
49768 DATA 174,255,207,202,142,255,207
49776 DATA 232,169,152,133,251,169,7
49784 DATA 133,252,56,165,251,233,80
49792 DATA 133,251,176,2,198,252,202
49800 DATA 208,242,160,0,177,251,201
49808 DATA 160,240,4,200,76,59,194
49816 DATA 174,251,207,169,32,145,251
49824 DATA 200,202,208,250,96,160,0
49832 DATA 152,153,0,212,200,192,9
49840 DATA 208,248,96,256
TI-99/4A Version Notes Patrick Parrish, Editorial Programmer Thanks to the outstanding sprite capabilities of Extended BASIC, the TI-99/4A version (Program 5) of "Diamond Drop" is a game with quick, smooth action. The object of the game is to catch colorful diamonds which fall from the top of the screen. You use a series of vertically positioned paddles. These paddles are controlled with the keyboard. We chose to use the S and D keys for left and right movement. However, if you are more comfortable using some other keys, simply substitute the ASCII values corresponding to the desired keys for the numbers 68 and 83 in lines 420 and 430. (To find the ASCII value of a key, use PRINT ASC("X"), where X is the key you want to use.) If you wish to use a joystick to play the game, change lines 420 to 440 to read: 420 CALL JOYST(1,H,V)::IF H=4 THEN H=60 430 IF H=-4 THEN H=-60 440 CALL MOTION(#1,0,H)::H=0::CALL JOYST( 1,H,V)::IF H=0 THEN CALL MOTION(#1,0 ,0) We have suggested these replacement lines, rather than incorporating both keyboard and joystick control into the game, because we found that the additional time required to execute a GOSUB in line 420 slightly slowed down the paddle response. There are two skill levels which are determined by how fast the diamonds drop. After you clear the entire screen of diamonds, the drop speed is increased. On the first screen, drop speed is 25 for skill level one, and 40 for skill level two. This is set in line 250. The drop speed is increased by three with completion of each screen in line 560. To make the game more challenging, the diamonds can be dropped along a random diagonal angle. With this feature, some interesting playing situations will develop. As screen wraparound of the paddles is permitted, you must often make quick decisions about which direction to move. A wrong move will ultimately affect your score since only ten misses are allowed. Scoring in the game, as determined in line 510, is affected by a number of factors. First, more points are awarded for diamonds garnered from successively higher rows on the screen. Second, diamond values increase with completion of each screen. Third, points are accumulated twice as quickly at skill level two. And last, if you choose to add an angle of descent to each diamond, a greater number of points are given based on the severity of the descent angle. When the game is over (when ten diamonds have been missed), your score and the high score for the session are posted. Extended BASIC for the TI-99/4A features some convenient commands for sprite manipulation. Since sprite movement can be very fast, detection of collisions between sprites is not always infallible. As noted in the TI Extended BASIC Manual, sprites which coincide in position can be detected only when the COINC subprogram is CALLed from BASIC. Thus, if your program is executing some statement other than CALL COINC when sprites cross, no collision will be detected. Fortunately, this is noticeable only at the most advanced levels in this game. |
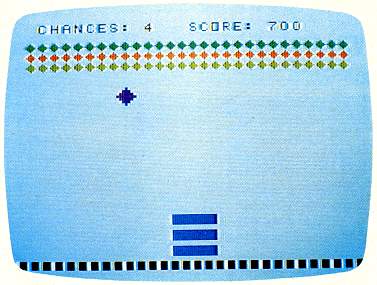
"Diamond Drop," TI version.
Program 5: Diamond Drop - TI-99/4A Version
by Patrick Parrish, Editorial Programmer
100 DIM KOLOR(6)
110 RANDOMIZE
120 GOSUB 630
130 REM 108-DEFINE DIAMOND SPRITE C
HAR,128-136 ARE THE PADDLES
140 CALL CHAR(108,"10387CFE7C381000
0000000000000000000000000000000
00000000000000000")
150 CALL CHAR(128,"FFFFFFFF0000FFFF
FFFF0000FFFFFFFFFFFFFFFF0000FFF
FFFFF0000FFFFFFFF")
160 CALL CHAR(132,"000000000000FFFF
FFFF0000FFFFFFFF000000000000FFF
FFFFF0000FFFFFFFF")
170 SCR=0 :: SK.=0 :: CH=10 :: S=0
: CALL CLEAR :: CALL SCREEN(16)
:: DISPLAY AT(4,9):"D I A M O N
D"
180 FOR ROW=3 TO 6
190 CALL HCHAR(ROW+2,6,32_,20)
200 DISPLAY AT(ROW+3,6):" ''' '''
{3 SPACES}'' '''" :: DISPLAY A
T(ROW+4,6):"h h h h h h h h
"
210 DISPLAY AT(ROW+5,6):"p p p p
p p p p" :: DISPLAY AT(ROW+6,
6):"x x xxx x x xxx"
220 DISPLAY AT(ROW+7,6):"h h h h
h h h" :: DISPLAY AT(ROW+8,6):
"''' ' ' '' '"
230 NEXT ROW
240 DISPLAY AT(18,4):"SKILL LEVEL
1,2) ?" :: ACCEPT AT(18,24)BEEP
VALIDATE("12")SIZE(1):SK$ :: S
K=VAL (SK".$ )
250 DROP=25 :: IF SK=2 THEN DROP=40
:: REM CHANGE DROP RATE TO CHA
NGE DIFFICULTY
260 DISPLAY AT(21,2):"DROP WITH ANG
LE (Y/N) ?" :: ACCEPT AT(21,26)
BEEP VALIDATE("YN")SIZE(1):ANG$
270 IF ANG$="N" THEN ANG=0 :: GOTO
290
280 ANG=1
290 CALL CLEAR :: SCR=SCR+1
300 DISPLAY AT(1,2):"CHANCES:";CH :
: DISPLAY AT(1,15):"SCORE:";S
310 ROW=3 :: FOR I=96 TO 120 STEP 8
320 CALL HCHAR(ROW,3,I,28):: ROW=RO
W+1 :: NEXT I
330 CALL HCHAR(24,1,30,32)
340 CALL MAGNIFY(4):: CALL SPRITE(#
1,128,5,150,115,0,H)
350 KHAR=108 :: ROW=41 :: FOR J=6 T
O 3 STEP -1
360 A$="" :: FOR I=3 TO 30 :: A$=A$
&CHR$(I):: NEXT I :: N=28
370 IF N=0 THEN 530
380 R=INT(LEN(A$)*RND+1):: P$=SEGO(
A$,R,1):: X=ASC(P$):: N=N-1 :.
IF N=0 THEN 400
390 A$=SEG$(A$,1,R-1)&SEG$(A$,R+1,L
EN(A$)-R)
400 B=INT(RND*61*ANG)-30*ANG
410 CALL HCHAR(J,X,32):: CALL SPRIT
E(#2,KHAR,KOLOR(J),ROW,8*(X-1)-
2,DROP,B)
420 CALL KEY(0,K,ST):: IF K=68 THEN
H=60 :: REM RIGHT MOVE-D KEY
430 IF K=83 THEN H=-60 :: REM LEFT
MOVE-S KEY
440 CALL MOTION(#1,0,H)::- H=0 :: CA
LL KEY(0,K,ST):: IF ST=0 THEN C
ALL MOTION(#1,0,0)
450 CALL COINC(ALL,C):: IF C THEN 5
10
460 CALL POSITION(#2,DROW,DCOL):: I
F DROW<155 THEN 420
470 CALL POSITION(#1,PROW,PCOL):: I
F (DCOL-PCOL=<16)*(DCOL-PCOL:=-8)
THEN 510
480 CALL DELSPRITE(#2):: CALL MOTIO
N(#1,0,0):: CH=CH-1 :: CALL SCR
EEN(11):: FOR F=0 TO 25 STEP 5
490 CALL SOUND(-200,-5,F):. NEXT F
:: CALL SCREEN(16):: IF CH=0 TH
EN GOTO 570
500 GOTO 520
510 CALL DELSPRITE(#2):: CALL MOTIO
N(#1,0,0):: S=S+(60/J)*SK*SCR+(
60/J)*SK*SCR*INT(ABS(B)/15)
520 DISPLAY AT(1,2):"CHANCES:";CH :
: DISPLAY AT(1_,15):"SCORE:";S
: GOTO 370
530 K=K+4 :: ROW=ROW-8 :: M=128 ::
IF J<6 THEN M=132
540 FOR F=0 TO 30 STEP 6 :: CALL SO
UND(-300,1500,F):: NEXT F
550 CALL SPRITE(#1,M,5,150,115,0,H)
560 NEXT J :: FOR G=600 TO 1400 STE
P 100 :: CALL SOUND(100,G,1)::
NEXT G :: DROP=DROP+3 :: GOTO 2
90
570 CALL SCREEN(14):: IF S>HS THEN
HS=S
580 CALL DELSPRITE(ALL):: CALL CLEA
R :: DISPLAY AT(8,5):"YOUR SCOR
E: ";S :: DISPLAY AT(11,5):"HIG
H SCORE: ";HS
590 DISPLAY AT(16,5):"PLAY AGAIN (Y
/N)? " :: ACCEPT AT(16,24)BEEP
VALIDATE("NY")SIZE(1):REPLY$
600 IF REPLY$="N" THEN 620
610 GOTO 170
620 STOP
630 REM DEFINE SMALL DIAMONDS AND C
OLORS
640 FOR I=96 TO 120 STEP 8
650 CALL CHAR (I,"10387CFE7C381000")
:: NEXT I
660 CALL COLOR(11,11,1)
670 CALL COLOR(9,3,1)
680 CALL COLOR(10,10,1)
690 CALL COLOR(12,14,1)
700 FOR J=3 TO 6 :: READ KOLOR(J)::
NEXT J
710 DATA 3,10,11,14
720 RETURN